翻譯自 這裡Preface :datetime 模組提供你方便的對日期與時間進行操作. 而更細微的實作與功能可以參考
time 與
calendar 模組. 另外在使用這裡的 date 與 time 物件時有區分為 "naive" 或 "aware" ; 關鍵就在於你有沒有將 Time Zone 的概念帶入. 事實上有一個屬性在負責這樣的資訊
tzinfo. 接著
datetime 模組有以下常數 :
- datetime.MINYEAR
The smallest year number allowed in a
date or
datetime object.
MINYEAR is 1.
- datetime.MAXYEAR
The largest year number allowed in a
date or
datetime object.
MAXYEAR is 9999.
Available Types :底下為
datetime 模組中支援的類別 :
- class datetime.date
An idealized naive date, assuming the current Gregorian calendar always was, and always will be, in effect. Attributes: year, month, and day.
- class datetime.time
An idealized time, independent of any particular day, assuming that every day has exactly 24*60*60 seconds (
there is no notion of “leap seconds” here). Attributes:
hour,
minute,
second,
microsecond, and
tzinfo.
- class datetime.datetime
A combination of a date and a time. Attributes:
year,
month,
day,
hour,
minute,
second,
microsecond, and
tzinfo.
- class datetime.timedelta
A duration expressing the difference between two
date,
time, or
datetime instances to microsecond resolution.
- class datetime.tzinfo
An abstract base class for time zone information objects. These are used by the
datetime and
time classes to provide a customizable notion of time adjustment (
for example, to account for time zone and/or daylight saving time).
上述類別建立的物件都是 immutable. 物件
date 一定是 naive ; 而由類別
time 或
datetime 建立的物件可能是 naive 或 aware (
如果物件上的 tzinfo 屬性不是 None, 則為 aware, 反之為 naive). 而在類別
timedelta 所建立的物件並沒有所謂的 naive 或是 aware.
timedelta Objects :類別
timedelta 所建立的物件 , 代表的是兩個 dates 或是 times 彼此間的時間差. 一旦引入 模組 datetime, 你可以如下建立 timedelta 物件 :
- class datetime.timedelta([days[, seconds[, microse...inutes[, hours[, weeks]]]]]]])
All arguments are optional and default to 0. Arguments may be ints, longs, or floats, and may be positive or negative.
在這些參數中, 只有
days,
seconds 與
microseconds 會真正儲存在物件中, 而其他參數會從以下關係進行轉換 :
* A millisecond is converted to 1000 microseconds.
* A minute is converted to 60 seconds.
* An hour is converted to 3600 seconds.
* A week is converted to 7 days.
而上面三個參數 days, seconds 與 microseconds 有最大與最小值範圍 :
* 0 <= microseconds < 1000000
* 0 <= seconds < 3600*24 (the number of seconds in one day)
* -999999999 <= days <= 999999999
此類別支援以下操作 :
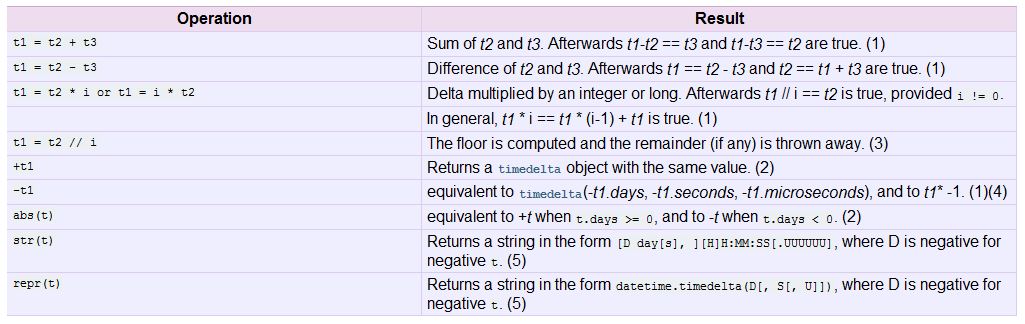
接著來看一些範例, 透過
timedelta 類別讓你可以對時間進行數學操作 :
>>> import datetime
>>> oneday = datetime.timedelta(1)
>>> thirtySec = datetime.timedelta(0, 30)
>>> tenMin = datetime.timedelta(0,0,0,0,10)
>>> td1 = oneday + tenMin # 1 天加 10分鐘
>>> print("One day + ten min = {0}".format(td1))
One day + ten min = 1 day, 0:10:00
>>> td2 = tenMin - thirtySec # 10分鐘 扣 30秒
>>> print("10 min - 30 sec = {0}".format(td2))
10 min - 30 sec = 0:09:30
>>> td3 = thirtySec * 6 # 6 個 30秒
>>> print("30 sec * 6 = {0}".format(td3))
30 sec * 6 = 0:03:00
>>> td4 = tenMin/10 # 10分鐘的 10分之一
>>> print("10 min / 10 = {0}".format(td4))
10 min / 10 = 0:01:00
timedelta 類別是
hashable ; 在 Boolean context 中, 只有當物件等於
timedelta(0) 才被視為 False, 除此之外都是 True. 除了上述支援的運算, 此類別還自帶一個方法 :
- timedelta.total_seconds()
New in version 2.7.
Return the total number of seconds contained in the duration. Equivalent to (td.microseconds + (td.seconds + td.days * 24 * 3600) * 10**6) / 10**6 computed with true division enabled.
>>> oneDay = datetime.timedelta(1)
>>> oneDay.total_seconds() # 一天共有 86400 秒
86400.0
接著來看一些整合的範例 :
date Objects :date 物件代表著日期包含年月日. 再引入模組
datetime 後你可以如下建立物件 :
- class datetime.date(year, month, day)
All arguments are required. Arguments may be ints or longs, in the following ranges :
* MINYEAR <= year <=
MAXYEAR* 1 <= month <= 12
* 1 <= day <= number of days in the given month and year
透過類別
date 的類別函數也可以方便的幫你建立物件 :
- classmethod date.today()
Return the current local date. This is equivalent to date.fromtimestamp(time.time()).
- classmethod date.fromtimestamp(timestamp)
Return the local date corresponding to the POSIX timestamp, such as is returned by
time.time(). This may raise
ValueError, if the timestamp is out of the range of values supported by the platform C localtime() function. It’s common for this to be restricted to years from 1970 through 2038. Note that on non-POSIX systems that include leap seconds in their notion of a timestamp, leap seconds are ignored by
fromtimestamp().
- classmethod date.fromordinal(ordinal)
Return the date corresponding to the
Proleptic Gregorian ordinal, where January 1 of year 1 has ordinal 1.
ValueError is raised unless
1 <= ordinal <= date.max.toordinal(). For any date
d,
date.fromordinal(d.toordinal()) == d.
本類別建立出來的物件支援以下操作 :

底下為簡單操作範例 :
>>> import datetime
>>> oneDay = datetime.timedelta(1)
>>> today = datetime.date.today()
>>> tomorrow = today + oneDay
>>> yesterday = today - oneDay
>>> "Today is {0}".format(today)
'Today is 2012-03-27'
>>> "Tomorrow is {0}".format(tomorrow)
'Tomorrow is 2012-03-28'
>>> "Yesterday is {0}".format(yesterday)
'Yesterday is 2012-03-26'
>>> dlta = tomorrow - yesterday
>>> print("Tomorrow - Yesterday = {0}".format(dlta))
Tomorrow - Yesterday = 2 days, 0:00:00
date 物件可以當作
dictionary 的鍵值 ; 在 Boolean context, 所有
date 物件都被視為 True. 另外物件上也有方法如下 :
- date.replace(year, month, day)
Return a date with the same value, except for those parameters given new values by whichever keyword arguments are specified. For example, if d == date(2002, 12, 31), then d.replace(day=26) == date(2002, 12, 26).
>>> today = datetime.date.today()
>>> print("Today is {0}".format(today))
Today is 2012-03-27
>>> otherDay = today.replace(day=1)
>>> print("Other day is {0}".format(otherDay))
Other day is 2012-03-01
- date.timetuple()
Return a
time.struct_time such as returned by
time.localtime(). The hours, minutes and seconds are 0, and the DST flag is -1.
d.timetuple() is equivalent to
time.struct_time((d.year, d.month, d.day, 0, 0, 0, d.weekday(), yday, -1)), where
yday = d.toordinal() - date(d.year, 1, 1).toordinal() + 1 is the day number within the current year starting with 1 for January 1st.
- date.toordinal()
Return the proleptic Gregorian ordinal of the date, where January 1 of year 1 has ordinal 1. For any
date object
d,
date.fromordinal(d.toordinal()) == d.
- date.weekday()
Return the day of the week as an integer, where
Monday is 0 and Sunday is 6. For example,
date(2002, 12, 4).weekday() == 2, a Wednesday. See also
isoweekday().
- date.isoweekday()
Return the day of the week as an integer, where
Monday is 1 and Sunday is 7. For example,
date(2002, 12, 4).isoweekday() == 3, a Wednesday. See also
weekday(),
isocalendar().
- date.isocalendar()
- date.isoformat()
Return a string representing the date in
ISO 8601 format, ‘YYYY-MM-DD’. For example,
date(2002, 12, 4).isoformat() == '2002-12-04'.
- date.__str__()
For a date d, str(d) is equivalent to d.isoformat().
- date.ctime()
Return a string representing the date, for example date(2002, 12, 4).ctime() == 'Wed Dec 4 00:00:00 2002'. d.ctime() is equivalent totime.ctime(time.mktime(d.timetuple())).
- date.strftime(format)
接著來看一些組合的範例 :
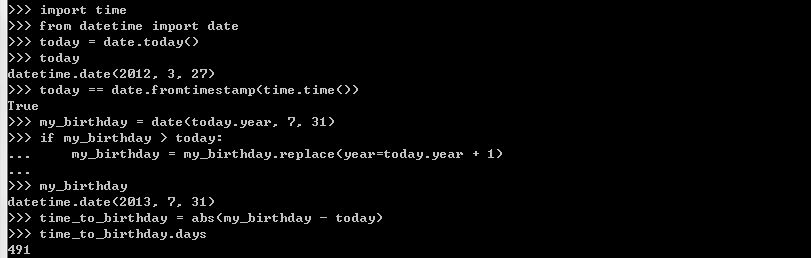
接著是一些取出
date 資訊的範例 :
datetime Objects :datetime 物件同時包含了物件
date 與 物件
time 所擁有的資訊. 你可以透過下面的建構子創建物件 :
- class datetime.datetime(year, month, day[, hour[, minute[, second[, microsecond[, tzinfo]]]]])
The
year,
month and
day arguments are required. tzinfo may be None, or an instance of a
tzinfo subclass. The remaining arguments may be ints or longs, in the following ranges :
* MINYEAR <= year <=
MAXYEAR* 1 <= month <= 12
* 1 <= day <= number of days in the given month and year
* 0 <= hour < 24
* 0 <= minute < 60
* 0 <= second < 60
* 0 <= microsecond < 1000000
If an argument outside those ranges is given,
ValueError is raised.
除此之外你也可以使用
datetime 上的類別方法建立物件 :
- classmethod datetime.today()
Return the current local datetime, with
tzinfo None. This is equivalent to
datetime.fromtimestamp(time.time()). See also
now(),
fromtimestamp().
- classmethod datetime.now([tz])
Return the current local date and time. If optional argument
tz is None or not specified, this is like
today(), but, if possible, supplies more precision than can be gotten from going through a
time.time() timestamp.
- classmethod datetime.utcnow()
Return the current
UTC date and time, with
tzinfo None. This is like
now(), but returns the current UTC date and time, as a naive
datetime object.
- classmethod datetime.fromtimestamp(timestamp[, tz])
Return the local date and time corresponding to the POSIX timestamp, such as is returned by
time.time(). If optional argument tz is None or not specified, the timestamp is converted to the platform’s local date and time, and the returned
datetime object is naive.
- classmethod datetime.utcfromtimestamp(timestamp)
Return the UTC
datetime corresponding to the POSIX timestamp, with
tzinfo None. This may raise
ValueError, if the timestamp is out of the range of values supported by the platform C gmtime() function. It’s common for this to be restricted to years in 1970 through 2038.
- classmethod datetime.fromordinal(ordinal)
Return the
datetime corresponding to the proleptic Gregorian ordinal, where January 1 of year 1 has ordinal 1.
ValueError is raised unless
1 <= ordinal <= datetime.max.toordinal(). The hour, minute, second and microsecond of the result are all 0, and
tzinfo is None.
- classmethod datetime.combine(date, time)
Return a new
datetime object whose date components are equal to the given
date object’s, and whose time components and
tzinfo attributes are equal to the given
time object’s. For any
datetime object
d,
d == datetime.combine(d.date(), d.timetz()). If
date is a
datetime object, its time components and
tzinfo attributes are ignored.
- classmethod datetime.strptime(date_string, format)
New in version 2.5.Return a datetime corresponding to date_string, parsed according to format. See
section strftime() and strptime() Behavior for more information.
>>> d = datetime.datetime.strptime('12:10:30',"%H:%M:%S") # 別忘記 import datetime>>> d.__str__()'1900-01-01
12:10:30'
建立完
datetime 物件後, 你可以透過該物件的屬性來讀取特定單位值 :
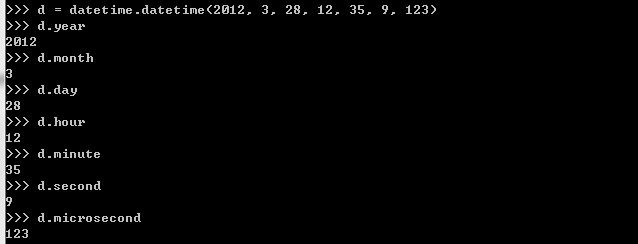
同樣你也可以對它下表運算 :
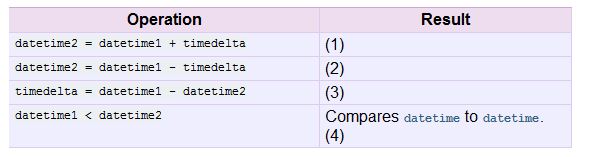
物件
datetime 可以當作 dictionary 的鍵值 ; 在 Boolean context 中, 所有
datetime 物件都被視為 True ; 底下是其物件上提供的方法 :
- datetime.date()
Return
date object with same year, month and day.
>>> d = datetime.datetime(2012, 3, 28, 12, 13, 14)>>> dt = d.date()>>> print("Date={0}".format(dt))Date=2012-03-28
- datetime.time()
Return
time object with same hour, minute, second and microsecond.
tzinfo is None.
>>> d = datetime.datetime(2012, 3, 28, 12, 13, 14)>>> print("Time={0}".format(d.time()))Time=12:13:14
- datetime.timetz()
Return
time object with same hour, minute, second, microsecond, and
tzinfo attributes.
- datetime.replace([year[, month[, day[, hour[, minute[, second[, microsecond[, tzinfo]]]]]]]])
Return a datetime with the same attributes, except for those attributes given new values by whichever keyword arguments are specified. Note that tzinfo=Nonecan be specified to create a naive datetime from an aware datetime with no conversion of date and time data.
- datetime.toordinal()
Return the proleptic Gregorian ordinal of the date. The same as self.date().toordinal().
- datetime.weekday()
Return the day of the week as an integer, where
Monday is 0 and Sunday is 6. The same as
self.date().weekday(). See also
isoweekday(),
isocalendar().
- datetime.isoweekday()
Return the day of the week as an integer, where Monday is 1 and Sunday is 7. The same as self.date().isoweekday().
- datetime.isocalendar()
Return a 3-tuple, (ISO year, ISO week number, ISO weekday). The same as self.date().isocalendar().
- datetime.isoformat([sep])
Return a string representing the date and time in ISO 8601 format, YYYY-MM-DDTHH:MM:SS.mmmmmm or, if microsecond is 0, YYYY-MM-DDTHH:MM:SS. The optional argument sep (default ' ') is a one-character separator, placed between the date and time portions of the result. For example :
>>> d = datetime.datetime(2002, 12, 25)
>>> d.isoformat('=')
'2002-12-25=00:00:00'
- datetime.strftime(format)
下面為
datetime 的組合範例 :
time Objects :time 物件代表一天的時間包括 時, 分, 秒等. 可以透過
tzinfo 對不同時區進行調整. 該類別的建構子如下 :
- class datetime.time(hour[, minute[, second[, microsecond[, tzinfo]]]])
All arguments are optional.
tzinfo may be None, or an instance of a
tzinfo subclass. The remaining arguments may be ints or longs, in the following ranges :
* 0 <=
hour < 24
* 0 <=
minute < 60
* 0 <=
second < 60
* 0 <=
microsecond < 1000000.
If an argument outside those ranges is given,
ValueError is raised. All default to 0 except tzinfo, which defaults to None.
在物件上提供方法如下 :
- time.replace([hour[, minute[, second[, microsecond[, tzinfo]]]]])
Return a
time with the same value, except for those attributes given new values by whichever keyword arguments are specified. Note that
tzinfo=None can be specified to create a naive
time from an aware
time, without conversion of the time data.
- time.isoformat()
Return a string representing the time in ISO 8601 format,
HH:MM:SS.mmmmmm or, if
self.microsecond is 0,
HH:MM:SS If
utcoffset() does not return None, a 6-character string is appended, giving the UTC offset in (
signed) hours and minutes:
HH:MM:SS.mmmmmm+HH:MM or, if self.microsecond is 0,
HH:MM:SS+HH:MM
- time.strftime(format)
- time.dst()
If
tzinfo is None, returns None, else returns
self.tzinfo.dst(None), and raises an exception if the latter doesn’t return None, or a
timedelta object representing a whole number of minutes with magnitude less than one day.
- time.tzname()
If
tzinfo is None, returns None, else returns
self.tzinfo.tzname(None), or raises an exception if the latter doesn’t return None or a string object.
底下為簡單使用範例 :
Supplement :*
Data Types : datetime - tzinfo Objects
tzinfo is an abstract base class, meaning that this class should not be instantiated directly. You need to derive a concrete subclass, and (
at least) supply implementations of the standard
tzinfo methods needed by the
datetime methods you use. The
datetime module does not supply any concrete subclasses of
tzinfo.
*
Data Types : calendar — General calendar-related functions
This module allows you to output calendars like the Unix
cal program, and provides additional useful functions related to the calendar. By default, these calendars have Monday as the first day of the week, and Sunday as the last (
the European convention). Use
setfirstweekday() to set the first day of the week to Sunday (6) or to any other weekday. Parameters that specify dates are given as integers. For related functionality, see also the
datetime and
time modules.