前言 :
在 Head-First Design Pattern 第二章接著介紹 Observer Pattern, 首先我們來看看它是怎麼介紹這個Pattern :
另外這裡也有提出一些設計原則 :
* Design Principe
Observer Pattern 定義 :
UML 示意圖 :

範例說明 :
在書中, 作者舉了一個氣象局RSS 的例子, 考慮以有一個WeatherData 物件可以提供相關氣象訊息, 並且它提供一支API:measurementsChanged 當氣象數據變更時, 這支API 就會被Callback. 而我們要作的就是當Callback 時, 則取出變化的氣象數據並顯示到Displays 上 (Display 可以是多個). 粗略示意圖如下 :
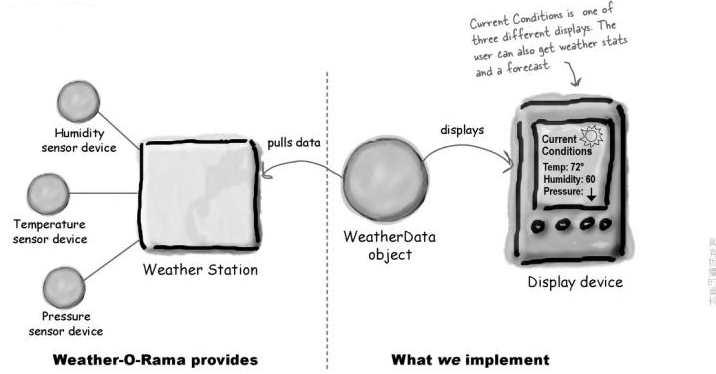
為了能夠動態增加Display devices 而在遵守設計原則 "OCP" (對修改封閉;對擴充開放), 可以聯想到報紙訂閱的流程 :
如果你懂怎麼訂閱報紙, 那麼觀察者模式就是 :
Publishers + Subscribers = Observer Pattern
其中publisher 就是 SUBJECT, 而 subscribers 就是 OBSERVERS.
範例代碼 :
請參考下面 UML 示意圖 : (詳細代碼請參考附件)

* WeatherStation 類別代碼 :
執行結果 :
補充說明 :
* Wiki on Observer Pattern :
* 史帝芬心得筆記 : Design Pattern - Observer
在 Head-First Design Pattern 第二章接著介紹 Observer Pattern, 首先我們來看看它是怎麼介紹這個Pattern :
另外這裡也有提出一些設計原則 :
* Design Principe
Observer Pattern 定義 :
UML 示意圖 :
範例說明 :
在書中, 作者舉了一個氣象局RSS 的例子, 考慮以有一個WeatherData 物件可以提供相關氣象訊息, 並且它提供一支API:measurementsChanged 當氣象數據變更時, 這支API 就會被Callback. 而我們要作的就是當Callback 時, 則取出變化的氣象數據並顯示到Displays 上 (Display 可以是多個). 粗略示意圖如下 :
為了能夠動態增加Display devices 而在遵守設計原則 "OCP" (對修改封閉;對擴充開放), 可以聯想到報紙訂閱的流程 :
如果你懂怎麼訂閱報紙, 那麼觀察者模式就是 :
Publishers + Subscribers = Observer Pattern
其中publisher 就是 SUBJECT, 而 subscribers 就是 OBSERVERS.
範例代碼 :
請參考下面 UML 示意圖 : (詳細代碼請參考附件)
* WeatherStation 類別代碼 :
- package hf.dp.ch02;
- public class WeatherStation {
- public static void main(String args[]) {
- WeatherData wd = new WeatherData(); //Create WeatherData object (Subject)
- CurrentConditionDisplay ccDisplay = new CurrentConditionDisplay(wd); //Create display element (Observer)
- wd.setMeasurement(55, 90, 12); // State change.
- wd.setMeasurement(60, 89, 15); // State change.
- ccDisplay.unSubscribe(); //Unsubscribe. So won't get updated from WeatherData.
- wd.setMeasurement(71, 92, 17); // State change.
- }
- }
執行結果 :
補充說明 :
* Wiki on Observer Pattern :
* 史帝芬心得筆記 : Design Pattern - Observer
Filename | hf.rar | |
Description | WeatherData 範例程式 | |
Filesize | 2 Kbytes | |
Downloaded: | 0 time(s) |