Source From Here
Preface
This post is to introduce an easy way to use personal access token with git (GitHub, GitLab, Bitbucket or whatever), such that you don’t have to enter your username and password when you push any commit. The only usage of personal access token in this post is to authorize git operations, like git push. Other usages are not discussed. I’m going to use osti@gitlab as an example. Other websites should be similar.
Generating a personal access token
Go to "Your Profile" > "Developer Settings" > "Personal access tokens" and Click on "Generate new token" to produce your personal token:
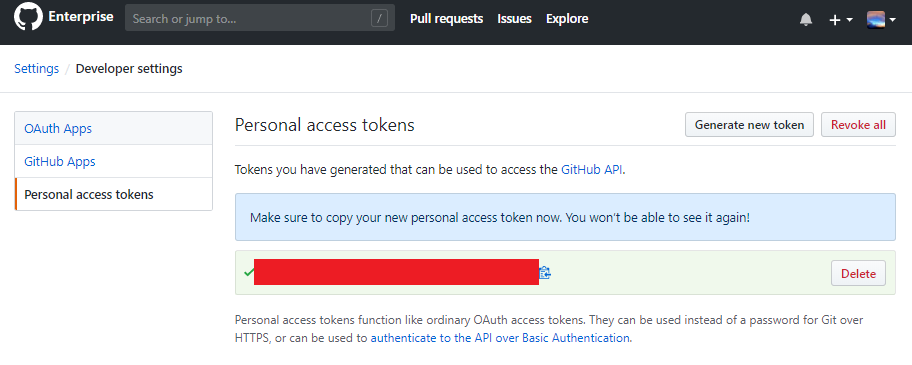
Save the new token for later authorization.
Using your token
Below is one example in using generated token above to do the authorization:
cxzhu:~/Documents/gits/test_at_osti$ git push origin master
But it is not a good idea to type the token everytime, especially it is a nonsense combination of characters.
Saving your token
The clever way is to save your personal access token by using curl, or other tools like git credentials. Here I’m going to introduce the easiest way using curl. I got the idea from here.
1. Save your token with curl
2. Add/replace your repository with authorizations You can clone a repository with authorization, like
Using ssh keys
When I use GitHub, I prefer to use ssh keys. You can find a detailed instruction on GitHub Help. It’s the most convenient and safest way. There are only two tips I would like to share.
1. Using ssh keys on MAC
To successfully add the key to ssh-agent, you can follow this page . But I found there is one additional step, at least on my macbook. That is you have to manually add github.com in known_hosts file, by typing:
2. Using ssh keys on Linux
On the GitHub Help Linux page, all the commands are suitable for bash shell. But if you are using other shells, like I use TC-shell on PPPL cluster, you have to replace the last two commands with the following ones.
Supplement
* FAQ - Where to store the personal access token from GitHub?
Preface
This post is to introduce an easy way to use personal access token with git (GitHub, GitLab, Bitbucket or whatever), such that you don’t have to enter your username and password when you push any commit. The only usage of personal access token in this post is to authorize git operations, like git push. Other usages are not discussed. I’m going to use osti@gitlab as an example. Other websites should be similar.
Generating a personal access token
Go to "Your Profile" > "Developer Settings" > "Personal access tokens" and Click on "Generate new token" to produce your personal token:
Save the new token for later authorization.
Using your token
Below is one example in using generated token above to do the authorization:
cxzhu:~/Documents/gits/test_at_osti$ git push origin master
- Username for 'https://gitlab.osti.gov': czhu
- Password for 'https://czhu@gitlab.osti.gov': yLVVsT8PbM-zxhrFRryM
- warning: redirecting to https://gitlab.osti.gov/czhu/test_at_osti.git/
- Counting objects: 3, done.
- Writing objects: 100% (3/3), 236 bytes | 236.00 KiB/s, done.
- Total 3 (delta 0), reused 0 (delta 0)
- To http://gitlab.osti.gov/czhu/test_at_osti.git
- * [new branch] master -> master
Saving your token
The clever way is to save your personal access token by using curl, or other tools like git credentials. Here I’m going to introduce the easiest way using curl. I got the idea from here.
1. Save your token with curl
2. Add/replace your repository with authorizations You can clone a repository with authorization, like
Using ssh keys
When I use GitHub, I prefer to use ssh keys. You can find a detailed instruction on GitHub Help. It’s the most convenient and safest way. There are only two tips I would like to share.
1. Using ssh keys on MAC
To successfully add the key to ssh-agent, you can follow this page . But I found there is one additional step, at least on my macbook. That is you have to manually add github.com in known_hosts file, by typing:
2. Using ssh keys on Linux
On the GitHub Help Linux page, all the commands are suitable for bash shell. But if you are using other shells, like I use TC-shell on PPPL cluster, you have to replace the last two commands with the following ones.
Supplement
* FAQ - Where to store the personal access token from GitHub?